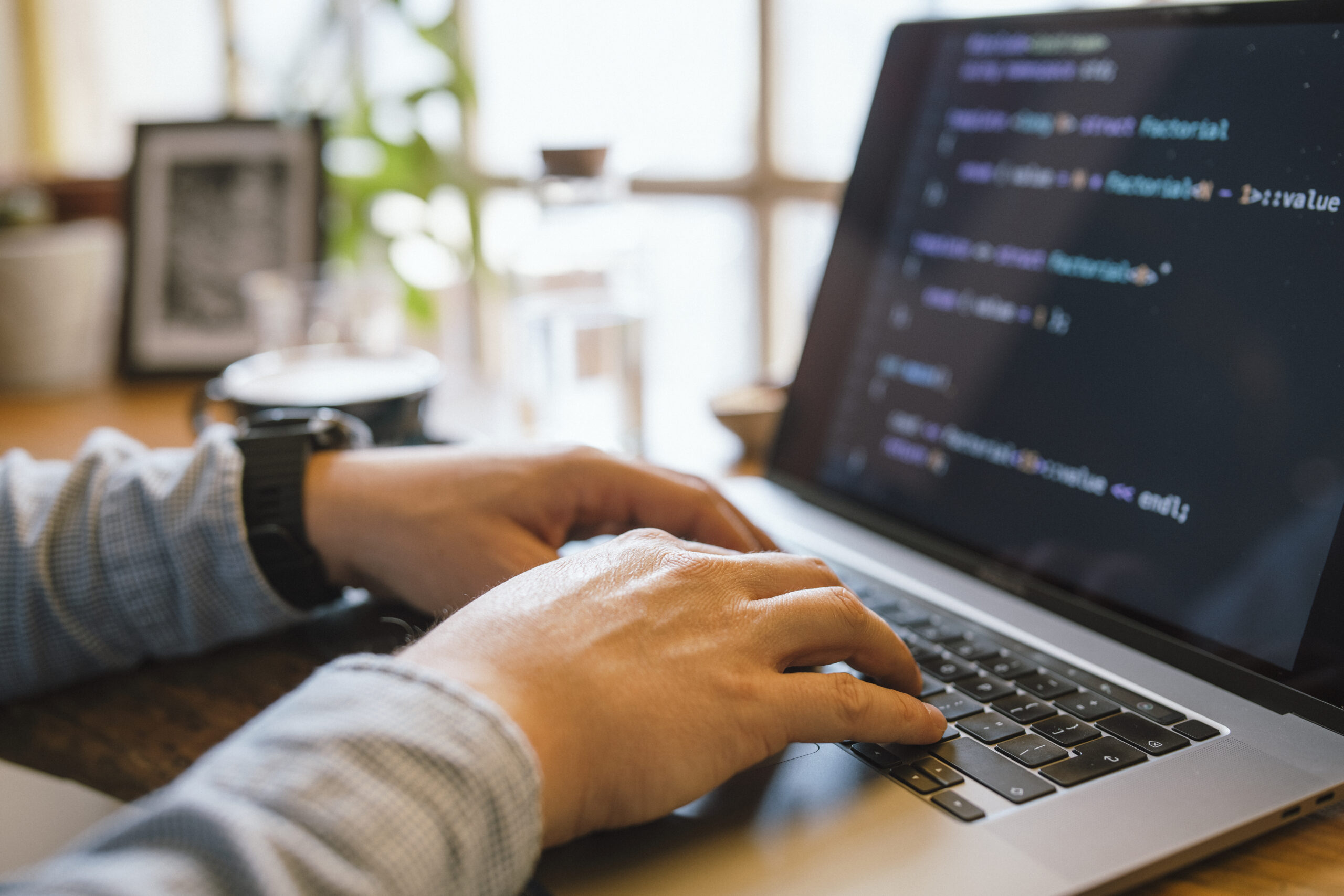
Debugging is Probably the most vital — nonetheless frequently disregarded — capabilities in a very developer’s toolkit. It isn't really just about fixing broken code; it’s about comprehending how and why factors go Completely wrong, and learning to think methodically to solve problems efficiently. Whether or not you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can conserve hrs of disappointment and substantially increase your productiveness. Listed below are numerous methods to assist builders amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest means builders can elevate their debugging capabilities is by mastering the resources they use each day. While crafting code is one particular Portion of improvement, knowing ways to communicate with it properly throughout execution is Similarly crucial. Modern enhancement environments appear equipped with impressive debugging capabilities — but many builders only scratch the surface of what these instruments can do.
Choose, by way of example, an Integrated Progress Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the value of variables at runtime, phase via code line by line, and in many cases modify code within the fly. When utilized appropriately, they Permit you to observe exactly how your code behaves through execution, which can be a must have for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, observe community requests, see serious-time functionality metrics, and debug JavaScript in the browser. Mastering the console, resources, and network tabs can convert irritating UI difficulties into workable tasks.
For backend or technique-amount developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle over working procedures and memory management. Understanding these instruments can have a steeper Understanding curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Over and above your IDE or debugger, come to be cozy with Variation Management units like Git to comprehend code historical past, come across the precise instant bugs had been introduced, and isolate problematic adjustments.
In the long run, mastering your applications indicates going past default settings and shortcuts — it’s about building an intimate familiarity with your growth natural environment to make sure that when challenges crop up, you’re not shed at the hours of darkness. The greater you are aware of your applications, the greater time you could shell out resolving the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the trouble
Just about the most vital — and often overlooked — actions in effective debugging is reproducing the problem. Right before leaping to the code or producing guesses, developers have to have to make a steady atmosphere or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug will become a match of opportunity, often bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Ask issues like: What actions led to The problem? Which atmosphere was it in — enhancement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it becomes to isolate the precise conditions underneath which the bug occurs.
When you finally’ve collected plenty of details, seek to recreate the trouble in your neighborhood surroundings. This may imply inputting a similar info, simulating identical user interactions, or mimicking technique states. If the issue appears intermittently, look at composing automatic tests that replicate the edge scenarios or state transitions concerned. These checks not only support expose the problem and also prevent regressions Sooner or later.
Sometimes, The problem can be environment-certain — it would materialize only on particular working devices, browsers, or less than specific configurations. Employing applications like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the problem isn’t only a phase — it’s a frame of mind. It involves tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible state of affairs, you can use your debugging tools much more successfully, check prospective fixes properly, and connect additional Plainly with the staff or people. It turns an summary grievance into a concrete challenge — Which’s where by developers prosper.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most respected clues a developer has when some thing goes wrong. Rather than looking at them as discouraging interruptions, builders must discover to take care of mistake messages as direct communications in the procedure. They generally inform you just what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start off by studying the information meticulously and in full. Lots of developers, especially when underneath time stress, look at the primary line and instantly get started generating assumptions. But deeper from the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines — read through and comprehend them initially.
Break the mistake down into components. Could it be a syntax error, a runtime exception, or possibly a logic mistake? Does it position to a specific file and line variety? What module or function induced it? These questions can tutorial your investigation and stage you toward the liable code.
It’s also useful to know the terminology with the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Discovering to recognize these can substantially increase your debugging method.
Some glitches are imprecise or generic, and in Individuals scenarios, it’s crucial to examine the context through which the mistake happened. Verify relevant log entries, enter values, and up to date modifications inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater difficulties and supply hints about potential bugs.
In the end, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, cut down debugging time, and turn into a more efficient and confident developer.
Use Logging Properly
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When applied correctly, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s going on beneath the hood while not having to pause execution or phase throughout the code line by line.
A superb logging approach begins with realizing what to log and at what degree. Typical logging amounts contain DEBUG, Information, WARN, Mistake, and Deadly. Use DEBUG for in depth diagnostic details in the course of improvement, INFO for typical situations (like prosperous start off-ups), WARN for more info potential challenges that don’t crack the appliance, ERROR for precise challenges, and FATAL when the process can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Far too much logging can obscure significant messages and slow down your system. Center on crucial occasions, point out adjustments, input/output values, and important selection points as part of your code.
Format your log messages Plainly and constantly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all with no halting This system. They’re Specially valuable in creation environments where by stepping by means of code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a effectively-considered-out logging approach, it is possible to lessen the time it will take to identify challenges, acquire deeper visibility into your apps, and Increase the overall maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a complex undertaking—it is a form of investigation. To efficiently discover and deal with bugs, builders must method the method just like a detective fixing a secret. This mentality helps break down complicated concerns into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the signs and symptoms of the issue: error messages, incorrect output, or functionality troubles. The same as a detective surveys a criminal offense scene, accumulate just as much applicable information and facts as you could without the need of leaping to conclusions. Use logs, exam scenarios, and person stories to piece jointly a clear image of what’s happening.
Next, form hypotheses. Talk to you: What can be resulting in this habits? Have any alterations not too long ago been produced towards the codebase? Has this issue happened in advance of beneath comparable circumstances? The intention will be to slim down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Make an effort to recreate the trouble in a managed surroundings. In the event you suspect a selected operate or component, isolate it and validate if The problem persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome lead you nearer to the truth.
Fork out close notice to modest particulars. Bugs generally conceal during the minimum envisioned areas—similar to a missing semicolon, an off-by-a person error, or simply a race problem. Be complete and individual, resisting the urge to patch The difficulty without having absolutely comprehension it. Temporary fixes could disguise the real challenge, only for it to resurface later on.
Last of all, hold notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging method can help you save time for long term troubles and help Other individuals have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical competencies, method challenges methodically, and become more effective at uncovering hidden troubles in elaborate methods.
Compose Assessments
Crafting checks is one of the most effective approaches to transform your debugging expertise and Total progress performance. Checks not just aid catch bugs early and also function a security Internet that offers you self esteem when earning changes for your codebase. A effectively-examined application is simpler to debug since it lets you pinpoint just wherever and when a challenge takes place.
Begin with device exams, which target specific features or modules. These tiny, isolated exams can swiftly reveal whether or not a specific piece of logic is Doing the job as envisioned. Every time a take a look at fails, you quickly know the place to search, substantially decreasing the time used debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear immediately after Earlier getting set.
Next, combine integration exams and end-to-close assessments into your workflow. These aid make sure that many portions of your application function alongside one another efficiently. They’re especially practical for catching bugs that arise in sophisticated systems with many elements or products and services interacting. If anything breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing tests also forces you to think critically regarding your code. To check a characteristic properly, you may need to know its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously prospects to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continually, you can target correcting the bug and watch your examination go when The difficulty is resolved. This technique makes certain that the identical bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from the disheartening guessing game into a structured and predictable approach—helping you catch a lot more bugs, speedier plus more reliably.
Consider Breaks
When debugging a tricky concern, it’s uncomplicated to be immersed in the condition—staring at your screen for hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging tools is simply stepping away. Taking breaks assists you reset your thoughts, decrease disappointment, and sometimes see the issue from a new perspective.
When you're as well close to the code for too long, cognitive fatigue sets in. You might start overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee break, or simply switching to a unique process for 10–15 minutes can refresh your aim. Lots of builders report locating the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist reduce burnout, In particular for the duration of lengthier debugging classes. Sitting down in front of a monitor, mentally caught, is not only unproductive and also draining. Stepping away allows you to return with renewed Electricity as well as a clearer mindset. You would possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you in advance of.
Should you’re trapped, a superb rule of thumb is usually to set a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It could feel counterintuitive, In particular under restricted deadlines, however it essentially results in more rapidly and more effective debugging Eventually.
To put it briefly, using breaks will not be a sign of weakness—it’s a wise strategy. It provides your Mind House to breathe, improves your viewpoint, and allows you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—it's an opportunity to increase for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you something useful in case you make the effort to replicate and examine what went Mistaken.
Start out by inquiring you a few important concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code testimonials, or logging? The solutions typically reveal blind spots within your workflow or knowing and allow you to Create more robust coding practices relocating forward.
Documenting bugs may also be a great habit. Keep a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Everything you discovered. As time passes, you’ll begin to see styles—recurring troubles or frequent blunders—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends might be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, supporting Other individuals steer clear of the similar concern boosts team effectiveness and cultivates a more powerful Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. All things considered, a few of the finest developers are certainly not the ones who produce ideal code, but people that constantly study from their errors.
In the long run, each bug you correct provides a fresh layer towards your skill set. So future time you squash a bug, take a second to replicate—you’ll come away a smarter, additional capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It can make you a far more economical, assured, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be better at Everything you do.